你真的需要Redux吗?
Redux 可帮助你处理共享状态的管理,但与任何工具一样,它也需要权衡利弊。使用 Redux 有更多的概念需要学习,还有更多的代码需要编写,需要添加了一些额外代码,并要求你遵循某些限制。这是短期和长期生产力之间的权衡。
在以下情况下使用 Redux:
应用中有很多 state 在多个组件中需要使用
应用 state 会随着时间的推移而频繁更新
更新 state 的逻辑很复杂
中型和大型代码量的应用,很多人协同开发
如果你的项目数据来源单一,没有过多的状态传递,也没有使用WebSocket等需要共享状态的功能,那么,Redux是不必须的。
Redux是什么
Redux 是一个使用叫做 “action” 的事件来管理和更新应用状态的模式和工具库 它以集中式 Store(centralized store)的方式对整个应用中使用的状态进行集中管理,其规则确保状态只能以可预测的方式更新。
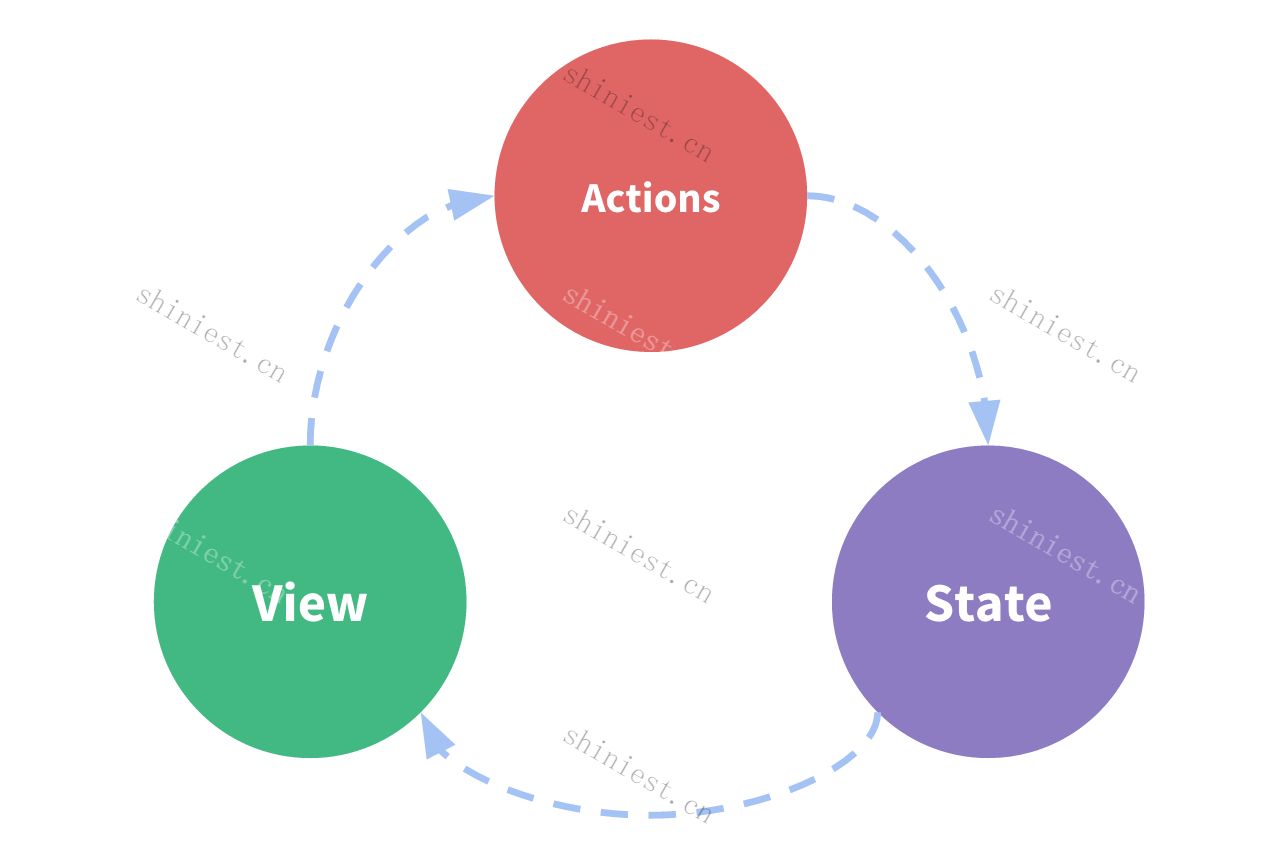
redux数据流
Redux架构原理:
剥离组件数据(state)
数据统一存放在store中
组件订阅store获得数据
store推送数据更新
类似与vue的状态管理vuex
一句话总结:Redux统一保存了数据,在隔离了数据与UI的同时,负责处理数据的绑定。
使用Redux
$ yarn add redux
# or
$ npm install redux --save
原则
store是唯一的 (整个应用只能有一个 store)
只有store能改变自己的内容 (store里的数据不是reducer更新的)
reducer必须是纯函数 (只要是同样的输入,必定得到同样的输出)
核心API
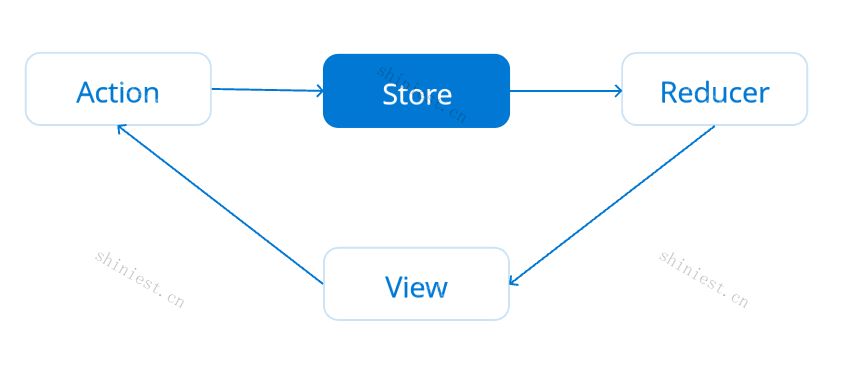
1. Store
Store 就是保存全局数据的地方,你可以把它看成一个容器(带有推送功能的数据仓库)。整个应用只能有一个 Store。
Redux 提供createStore
这个函数,用来生成 Store。
import { createStore } from 'redux';
const store = createStore(fn);
2. State
Store对象包含所有数据。如果想得到某个时点的数据,就要对 Store 生成快照。这种时点的数据集合,就叫做 State。
当前时刻的 State,可以通过store.getState()
拿到。
import { createStore } from 'redux';
const store = createStore(fn);
const state = store.getState();
Redux 规定, 一个 State 对应一个 View。只要 State 相同,View 就相同。你知道 State,就知道 View 是什么样,反之亦然。
3. Action
State 的变化,会导致 View 的变化。但是,用户接触不到 State,只能接触到 View。所以,State 的变化必须是 View 导致的。
Action 就是 View 发出的通知,表示 State 应该要发生变化了。
Action 是一个对象。其中的type属性是必须的,表示 Action 的名称。其他属性可以自由设置。
const action = {
type: 'indexChange',
value: 1,
};
上面代码中,Action 的名称是indexChange
,它携带的参数是boolean
类型的 1
可以这样理解,Action 描述当前发生的事情。改变 State 的唯一办法,就是使用 Action。它会运送数据到 Store。
4. Action Creator
过多的Action在组件中,会显得代码过于臃肿,不利于阅读和维护
可以新建一个actionCreator.js
文件,用来统一管理action
// action的统一管理
export const changeInputAction = value => ({
type: 'change_input',
value,
});
export const addItemAction = () => ({
type: 'add_item',
});
export const delItemAction = index => ({
type: 'del_item',
index,
});
export const initListAction = list => ({
type: 'init_list',
list,
});
每个函数都用来返回一个action,这个函数就叫 Action Creator。
5. store.dispatch()
store.dispatch()
是 View 发出 Action 的唯一方法。
import { createStore } from 'redux';
const store = createStore(fn);
const action = {
type: 'change_input',
value: 'Learn Redux',
};
store.dispatch(action);
结合 Action Creator
,这段代码可以改写如下:
import { createStore } from 'redux';
import { changeInputAction } from 'src/store/actionCreator.js'
const store = createStore(fn);
store.dispatch(changeInputAction('Learn Redux'));
6. Reducer
Store 收到 Action 以后,必须给出一个新的 State,这样 View 才会发生变化。这种 State 的计算过程就叫做 Reducer。
Reducer 是一个函数,它接受 Action 和当前 State 作为参数,返回一个新的 State。
可以新建一个reducer.js
文件,用来统一管理
src/store/reducer.js
// 初始状态,作为 State 的默认值
const defaultState = {
value: ''
};
const reducer = (state = defaultState, action) => {
let newState = JSON.parse(JSON.stringify(state)); // 深拷贝,不能直接修改state里的数据
if (action.type === 'change_input') {
newState.value = action.value;
}
if (action.type === 'add_item') {
newState.list.push(newState.value);
newState.value = '';
}
if (action.type === 'del_item') {
newState.list.splice(action.index, 1);
}
if (action.type === 'init_list') {
newState.list = action.list;
}
return newState;
};
export default reducer;
reducer函数
收到名为change_input
、add_item
、del_item
、init_list
的 Action 后,就返回一个新的 State(newState
),作为结果抛出。
在生成 Store 的时候,将 reducer
传入createStore()
。以后每当store.dispatch
发送过来一个新的 Action
,就会自动调用 reducer
,得到新的 State
。
import { createStore } from 'redux';
import reducer from 'src/store/reducer.js';
const store = createStore(reducer);
7. store.subscribe()
Store 允许使用store.subscribe()
方法设置监听函数,一旦 State 发生变化,就自动执行这个函数。
import { Component} from 'react';
import store from './store/index';
class List extends Component {
constructor(props) {
super(props);
store.subscribe(this.storeChange.bind(this)); // store发生改变时,自动触发storeChange方法
}
render() {
return //...
}
storeChange() {
// store发生改变时,将自动触发
let newState = store.getState(); // 得到当前state状态,包含所有store属性
this.setState(newState); // 重新渲染 View
}
}
export default List;
store.subscribe
方法返回一个函数,调用这个函数就可以解除监听。
let unsubscribe = store.subscribe(() =>
console.log(store.getState())
);
unsubscribe(); // 解除监听
工作流程
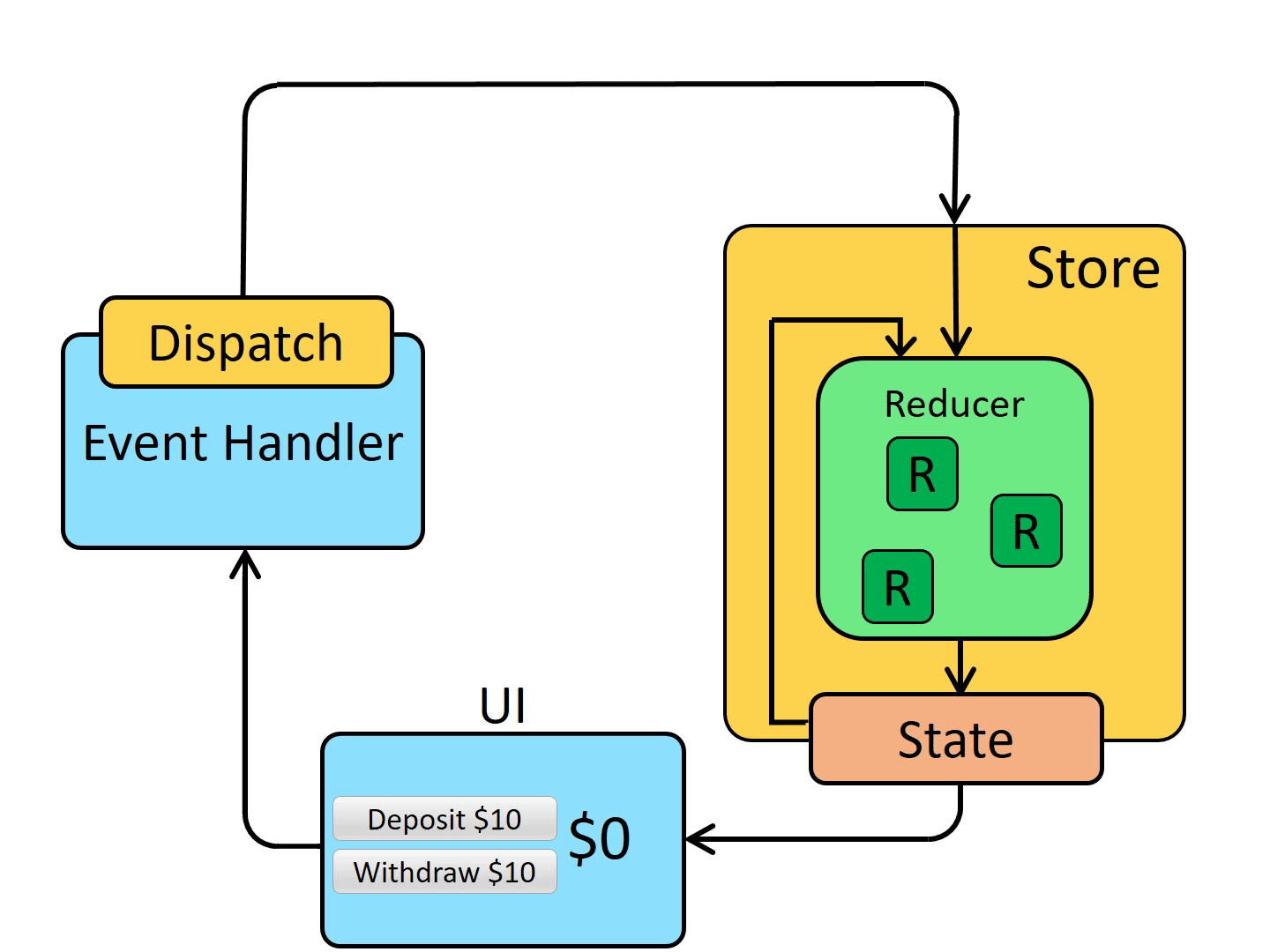
1. 用户发出 Action
const action = {
type: 'change_input',
value: 'Learn Redux',
};
store.dispatch(action);
2. Store 自动调用 Reducer,并且传入两个参数:当前 State 和收到的 Action。 Reducer 会返回新的 State(newState)
const reducer = (state, action) => {
let newState = JSON.parse(JSON.stringify(state)); // 深拷贝,不能直接修改state里的数据
if (action.type === 'change_input') {
newState.value = action.value;
}
return newState;
};
export default reducer;
3. State 一旦有变化,Store 就会调用监听函数
store.subscribe(this.storeChange.bind(this)); // store发生改变时,自动触发storeChange方法
4.storeChange()可以通过store.getState()得到当前状态。如果使用的是 React,这时可以触发重新渲染 View
storeChange() {
// store发生改变时,将自动触发
let newState = store.getState(); // 得到当前state状态,包含所有store属性
this.setState(newState); // 重新渲染 View
}
完整示例
src/store/index.js
import { createStore } from 'redux';
import reducer from './reducer';
const store = createStore(reducer, window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__ && window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__());
export default store;
src/list.js
import store from './store/index';
import { changeInputAction } from './store/actionCreator';
class List extends Component {
constructor(props) {
super(props);
this.state = store.getState(); // 获取store中所有的数据
store.subscribe(this.storeChange.bind(this)); // store发生改变时,自动触发
}
render() {
return (
<div>
<input value={this.state.value} onChange={this.change.bind(this)} />
</div>
);
}
storeChange() {
this.setState(store.getState());
}
change(e) {
const action = changeInputAction(e.target.value);
store.dispatch(action); // 派发action给store
}
}
export default List;
src/store/actionCreator.js
export const changeInputAction = value => ({
type: 'change_input',
value,
});
src/store/reducer.js
const defaultState = {
value: ''
};
const reducer = (state = defaultState, action) => {
console.log(state, action);
let newState = JSON.parse(JSON.stringify(state)); // 深拷贝,不能直接修改state里的数据
if (action.type === 'change_input') {
newState.value = action.value;
}
return newState;
};
export default reducer;